Allow Your Curiosity to Guide You on a Journey of Exploration and Learning.
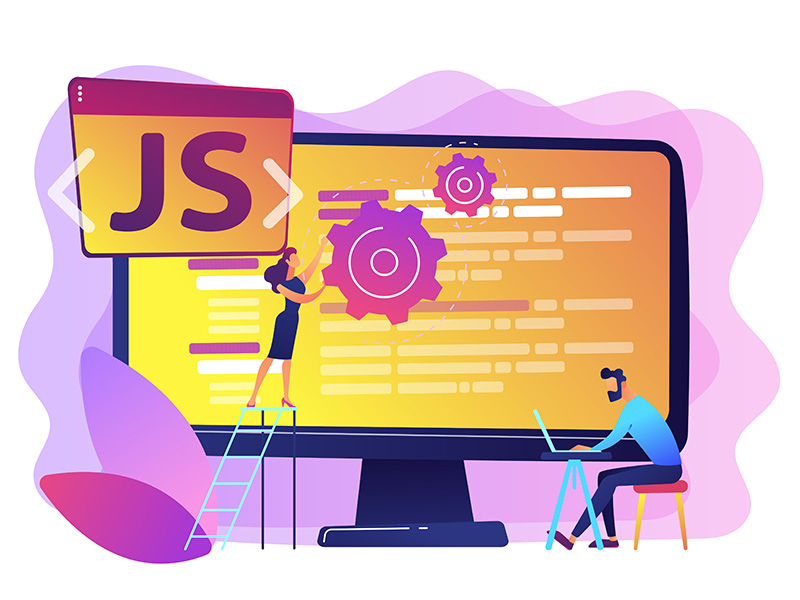
Events and Event Handling
Understanding how to handle user interactions and respond to events such as clicks, keypresses, and form submissions.
JavaScript, the language that breathes life into the web, empowers developers to create dynamic and interactive user experiences. At the core of this interactivity lies the concept of events and event handling. In this guide, we’ll explore the world of events, understanding how to handle user interactions and respond to events such as clicks, keypresses, and form submissions.
Understanding Events: The Pulse of User Interactions
In the web development landscape, events are moments when a user interacts with a webpage, triggering a specific action or response. Examples of events include clicking a button, pressing a key, or submitting a form. JavaScript enables developers to capture and respond to these events, adding a layer of interactivity to static web pages.
Event Handling: Capturing the Beat of User Actions
Event handling involves specifying code that should run in response to a particular event. In JavaScript, event handling is achieved by associating a function, known as an event handler, with a specific event.
1. Click Events: Adding Interactivity with Mouse Clicks
The click
event is one of the most common events, occurring when a user clicks an element on the page. Event handling for click events can be done with JavaScript.
// HTML: <button id="myButton">Click me</button>
let myButton = document.getElementById('myButton');
// JavaScript: Event handling for click
myButton.addEventListener('click', function() {
console.log('Button clicked!');
});
2. Keypress Events: Reacting to Keyboard Inputs
Keypress events allow you to capture and respond to key presses. This is useful for creating keyboard shortcuts or validating user input.
// JavaScript: Event handling for keypress
document.addEventListener('keypress', function(event) {
console.log('Key pressed: ' + event.key);
});
3. Form Submission Events: Processing User Input
Form submission events are crucial for handling user input and performing actions based on form submissions.
<!-- HTML: <form id="myForm"><input type="text" id="textInput"/><button type="submit">Submit</button></form> -->
// JavaScript: Event handling for form submission
let myForm = document.getElementById('myForm');
myForm.addEventListener('submit', function(event) {
event.preventDefault(); // Prevents the default form submission
let userInput = document.getElementById('textInput').value;
console.log('User input: ' + userInput);
});
Events and event handling in JavaScript are the orchestrators of interactivity on the web. By understanding how to capture and respond to events, developers can create engaging and responsive user interfaces. As you continue your coding journey, experiment with different events and explore the myriad ways they can enhance the user experience of your web applications.